記事内で紹介する商品を購入することで、当サイトに売り上げの一部が還元されることがあります。
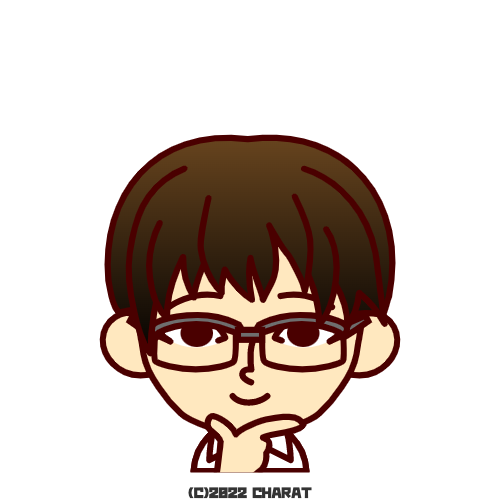
タイピングゲーム制作を通してプログラミングに強くなろう!
ということで、オリジナル 対戦型タイピングゲームの制作過程を紹介しています。
このタイピングゲームは
- HTML
- CSS
- JavaScript
の3種類の言語で制作していて初心者でも取り扱いやすい内容になっていて、
高校の情報1のプログラミング実技授業としてもオススメです!
この記事では制作過程の「Step1 基本的なHTML、CSS、JSの理解と入力時に文字が灰色になる機能の実装しよう」ということで、HTMLとCSS、JavaScriptファイルを更新して、攻撃のモーションを加えてみましょう!
目次
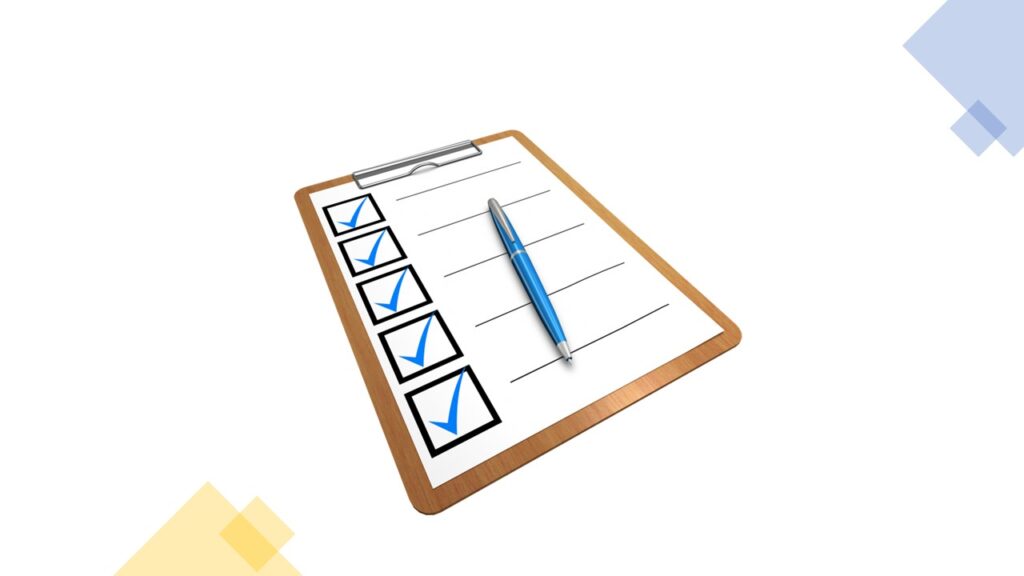
Step1では
- 基本的なHTML、CSS、JavaScriptの理解
- トップ画面で「ゲーム開始」を押すとバトル画面に遷移する機能の実装
- イベントリスナーとDOM操作の基礎を理解
- 正しく入力した際にリアルタイムで問題の文字が灰色になる機能の実装
これらを目標としています。
Step1を終えるには目安として1〜2時間必要です。
Step1で入力するコードで正しく機能を実装できれば以下のようなプログラムの状態になります。
【スタート画面】
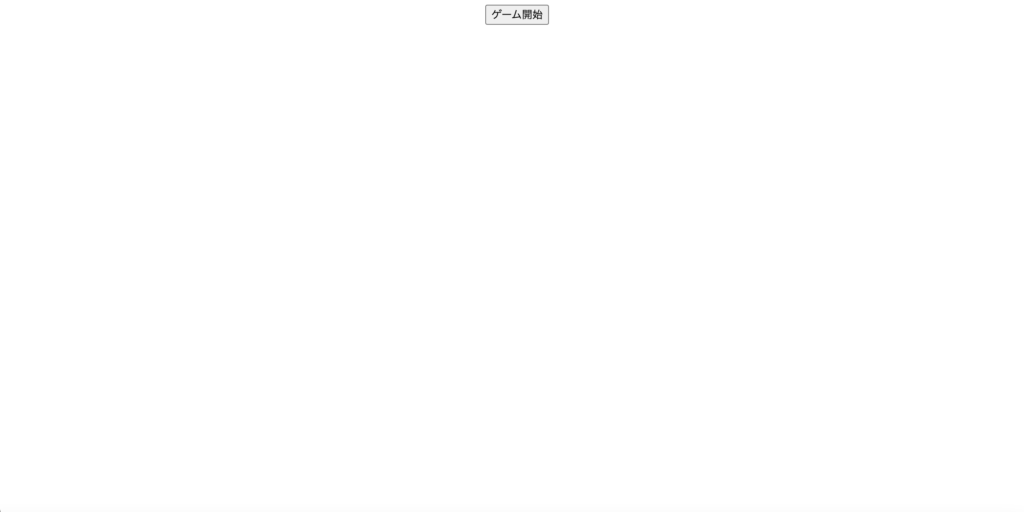
入力画面
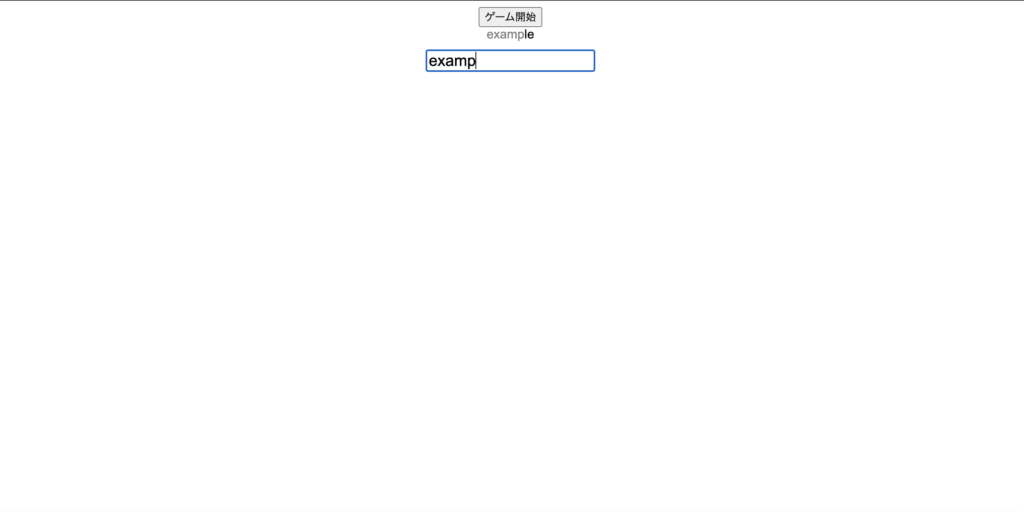
入力した文字があっていると、灰色になっていることが確認できます。
制作に必要なものも多くありません。
- パソコン
- インターネットに接続できる環境
- コードエディタ
これらを揃えましょう。
コードエディタは、VSCodeなど専門的なソフトがあれば良いですが、準備するのが難しい際には
などインターネット上で利用できるエディターを使用しましょう。
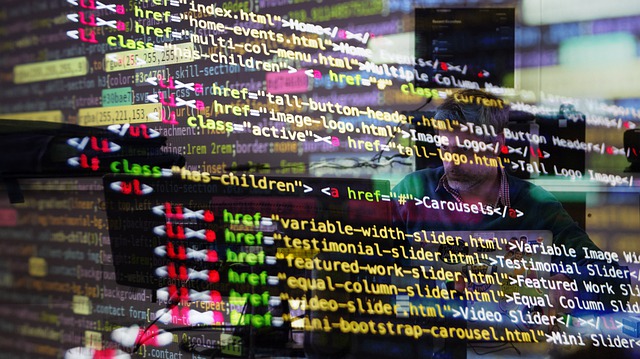
それではStep1をどのように進めていくか順に説明します。
授業では、下記の課題ファイルを準備し、上から順にコードを解説していきます。
特に初めなので、index.htmlの各タグを説明すると良いでしょう。
ただ、このStepではHTMLファイルの更新は不要でJavaScriptファイルのみ更新すればOKです。
ヒントを出しながら、課題のCSSファイルとJavaScriptファイルを更新しましょう。
この課題が完了すれば、入力された文字が合っていると灰色になることを確認しましょう。
JavaScriptファイルでgetNextWord()を完成させましょう。
ポイントは、以下の2点です。
- 配列を作る。好きな単語を10個ほど作成させ、「const words = [‘example’, ‘challenge’, ‘javascript’, ‘typing’, ‘game’];」のように単語を” “で囲って記述させる。
- ヒントを出しながら「return」「Math.floor()」「Math.random()」を使い「return words[Math.floor(Math.random() * words.length)];」と導き出させる。
ここまでを完了させれば、タイピング中の正誤判定機能と次の問題を表示する機能が実装できているはずです!
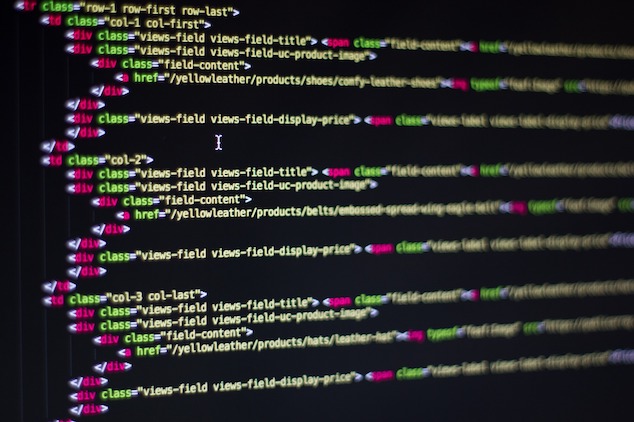
最後に模範コードと課題用コードの例を紹介します。
課題用コードの編集箇所は好きにアレンジしてください!
HTMLファイルの名前は「index.html」にしましょう。
模範コード
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>タイピングゲーム</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<button id="startButton">ゲーム開始</button>
<div id="game" style="display: none;">
<div id="wordDisplay"></div>
<input type="text" id="wordInput">
</div>
<script src="script.js"></script>
</body>
</html>
課題用コードの例
このStepではHTMLファイルは更新しませんので、模範コードを準備すればOKです。
HTMLファイルで読み込むCSSファイルの名称を指定しているので、「style.css」というファイル名にしておきましょう。
模範コード
/* style.css */
body {
font-family: Arial, sans-serif;
text-align: center;
}
#wordDisplay span.correct {
color: grey;
}
#wordInput {
margin: 10px;
font-size: 20px;
}
課題用コードの例
CSSファイルもHTMLファイル同様、このステップでは更新しませんので模範コード通りで準備しましょう。
JavaScriptのファイル名は「script.js」としておきましょう。
模範コード
document.addEventListener ('DOMContentLoaded' ,()=>{
const startButton = document.getElementById('startButton');
const game = document.getElementById('game');
const wordDisplay = document.getElementById('wordDisplay');
const wordInput = document.getElementById('wordInput');
let currentWord = 'example';
startButton.addEventListener('click', startGame);
wordInput.addEventListener ('input',checkInput);
function startGame(){
updateWordDisplay();
game.style.display = 'block';
wordInput.value ='';
wordInput.focus();
}
function updateWordDisplay(){
wordDisplay.innerHTML = currentWord.split('').map(char => `<span>${char}</span>`).join('');
}
function checkInput () {
const arrayWord = currentWord.split('');
const arrayInput = wordInput.value.split('');
arrayWord.forEach((char, index) => {
const charSpan = wordDisplay.children[index];
if(arrayInput[index] === char){
charSpan.classList.add('correct');
}else{
charSpan.classList.remove('correct');
}
});
if(wordInput.value === currentWord){
currentWord = getNextWord();
updateWordDisplay();
wordInput.value = '';
}
}
function getNextWord(){
const words = ['try', 'get', 'hello'];
return words[Math.floor(Math.random() * words.length)];
};
});
課題用コードの例
// DOMContentLoaded イベントが発生した後に実行される関数を設定
document.addEventListener('DOMContentLoaded', () => {
// HTML要素を取得
const startButton = document.getElementById('■■■■');
const game = document.getElementById('game');
const wordDisplay = document.getElementById('wordDisplay');
const wordInput = document.getElementById('■■■■■■■');
// 最初に表示する単語を設定
let currentWord = "example";
// イベントリスナーを追加
■■■.addEventListener('click', startGame); // ゲーム開始ボタンのクリックイベント
■■■.■■■■■■■■■■■■■■■■('input', checkInput); // 入力フィールドの入力イベント
// ゲームを開始する関数
function startGame() {
updateWordDisplay(); // 単語表示を更新
game.style.display = "block"; // ゲーム画面を表示
wordInput.value = ""; // 入力フィールドを空に
wordInput.focus(); // 入力フィールドにフォーカスを合わせる
}
// 単語表示を更新する関数
function updateWordDisplay() {
// 単語の各文字を分割し、それぞれの文字に対してspanタグを追加
wordDisplay.innerHTML = currentWord.split('').map(char => `<span>${char}</span>`).join('');
}
// 入力のチェックを行う関数
function checkInput() {
// 現在の単語と入力された文字を配列に変換
const arrayWord = currentWord.split('');
const arrayInput = wordInput.value.split('');
// 各文字をチェックし、正しい入力かどうか判定
arrayWord.■■■■■■■((char, index) => {
const charSpan = wordDisplay.children[index];
if (arrayInput[index] === char) {
charSpan.classList.add('correct'); // 正しい文字には 'correct' クラスを追加
} else {
charSpan.classList.remove('correct'); // 正しくない場合は 'correct' クラスを削除
}
});
// 全ての文字が正しく入力された場合
if (wordInput.value === currentWord) {
currentWord = ■■■(); // 次の単語を取得
■■■(); // 単語表示を更新
wordInput.value = ''; // 入力フィールドを空に
}
}
// 次の単語をランダムに取得する関数
function getNextWord() {
// 利用可能な単語のリスト
// リストからランダムに単語を選択して返す
}
});
Step1の詳細と進め方を紹介しました。
次はStep2「攻撃のモーションをつけよう」です。
次回もお楽しみに!
スポンサーリンク